https://pythonturtle.academy/tutorial-round-rectangle-or-square-with-python-turtle/
In this tutorial we are going to show how to draw rectangles or squares with round corners.
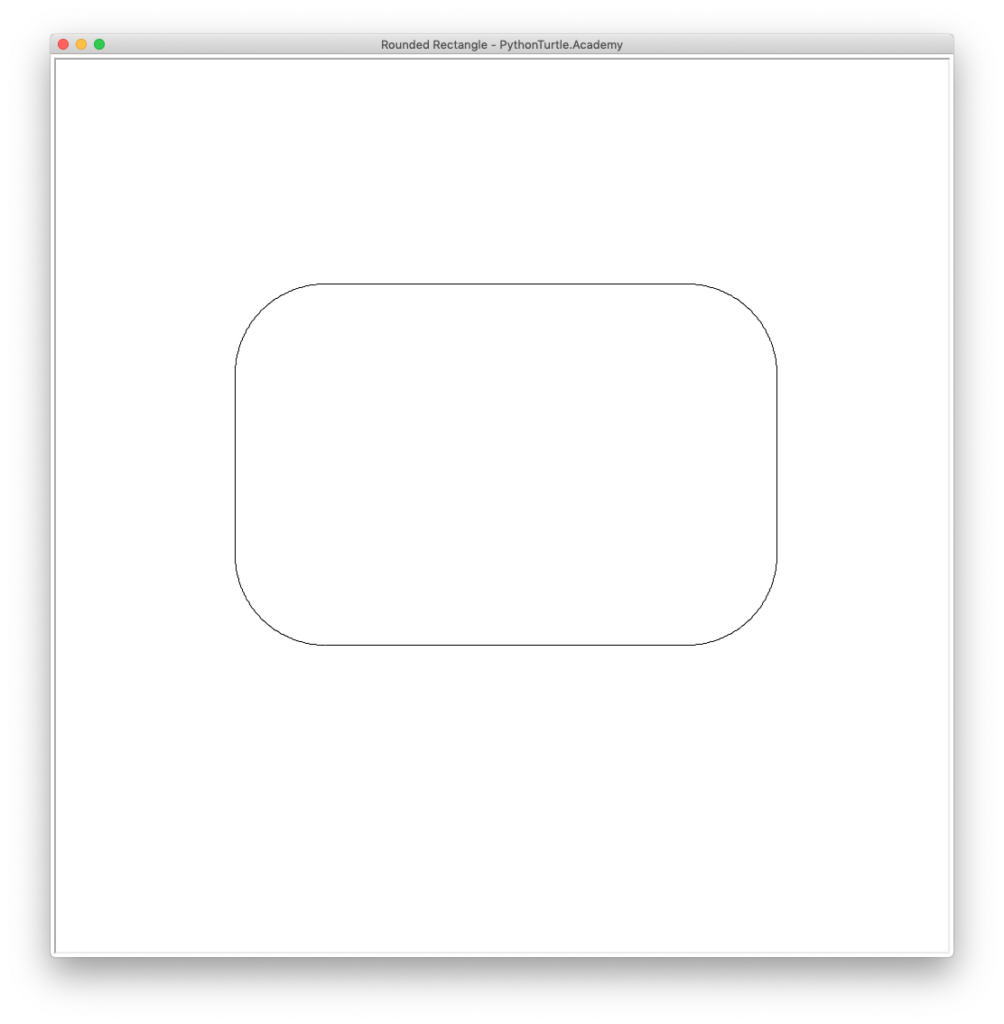
We will start by drawing the bottom straight-line with blue color. The following is the code snippet:
turtle.up()
turtle.goto(-200,-150)
turtle.color('blue')
turtle.down()
turtle.dot()
turtle.fd(400)
turtle.dot()
It should draw the following shape. The blue dots were drawn to show the starting and end points. They will be removed later.
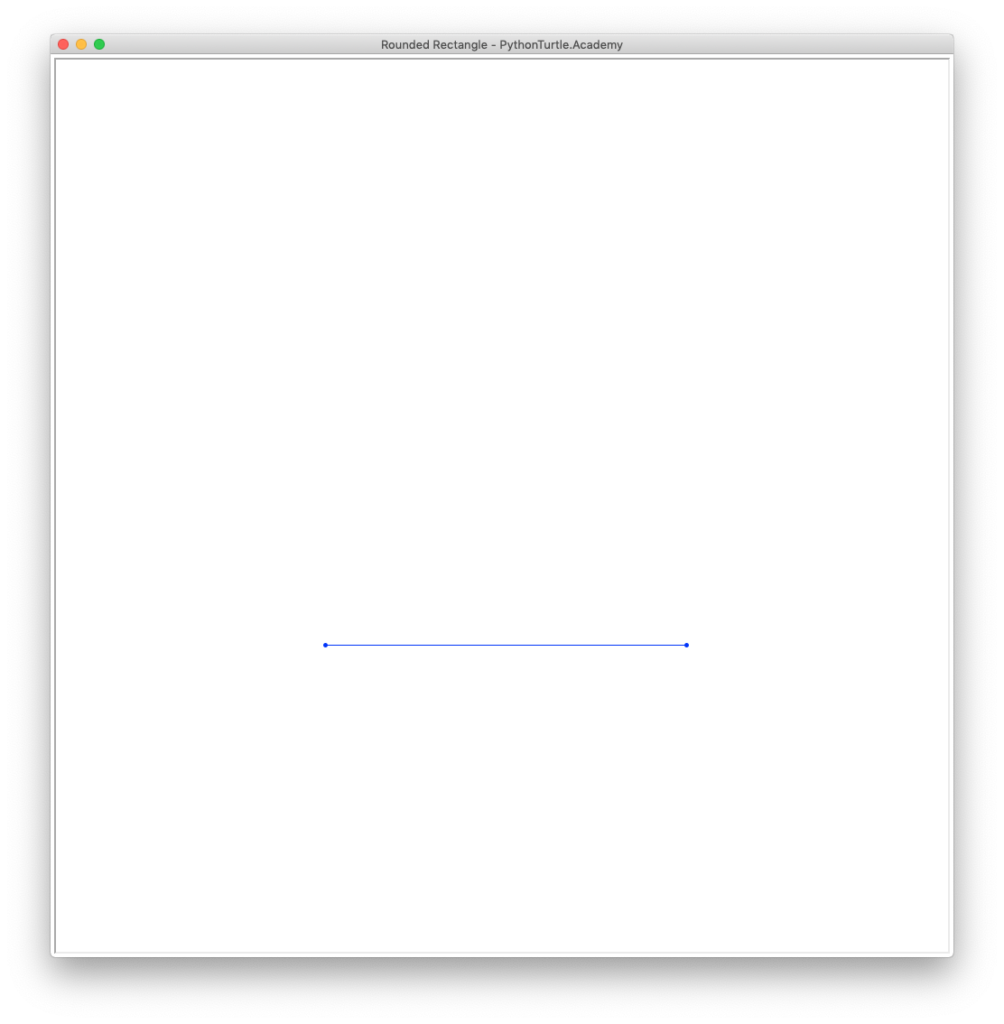
We are going to draw the round corners with 90 degree arc of a circle in red color. The radius of the arc can be any number. Smaller radius generates smaller round corners. The following is the code snippet for drawing the round corner:
turtle.up()
turtle.goto(-200,-150)
turtle.color('blue')
turtle.down()
turtle.dot()
turtle.fd(400)
turtle.dot()
turtle.color('red')
turtle.circle(100,90)
turtle.dot()
It should draw a shape like this:
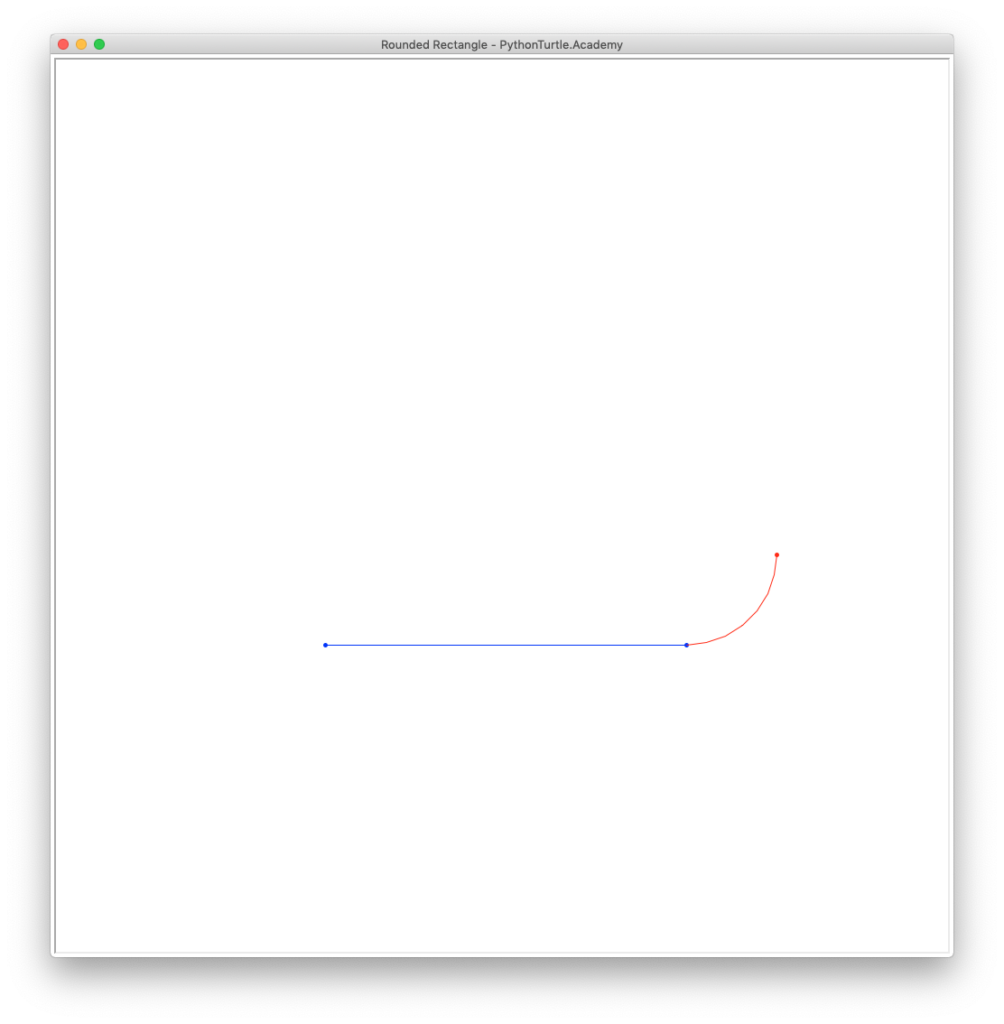
The next step will be easier. Just repeat the step above for the next side. The following is the code snippet:
turtle.up()
turtle.goto(-200,-150)
turtle.color('blue')
turtle.down()
turtle.dot()
turtle.fd(400)
turtle.dot()
turtle.color('red')
turtle.circle(100,90)
turtle.dot()
turtle.color('blue')
turtle.fd(200)
turtle.dot()
turtle.color('red')
turtle.circle(100,90)
turtle.dot()
It draws a shape like the following:
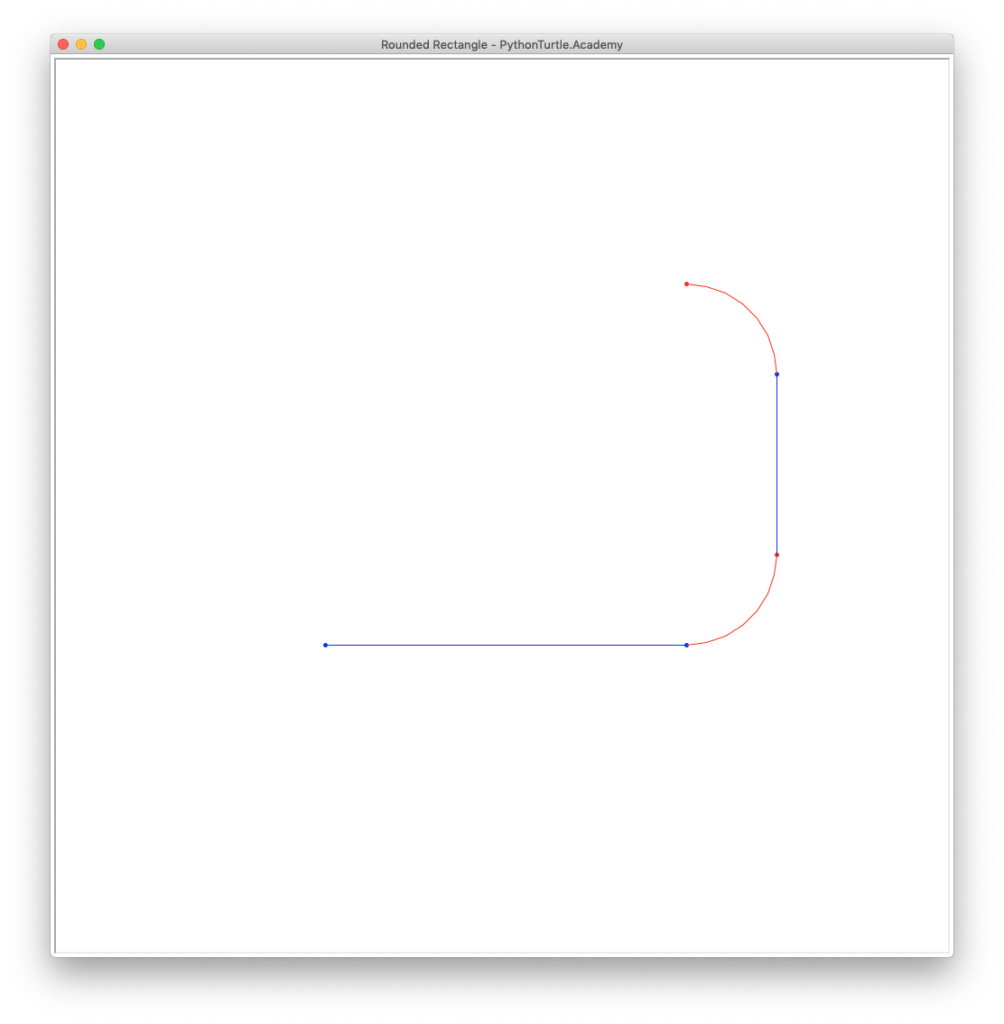
To finish the whole round rectangle, we just need to repeat the process one more time. The following is the complete code snippet with dots and colors removed:
turtle.up()
turtle.goto(-200,-150)
turtle.down()
for _ in range(2):
turtle.fd(400)
turtle.circle(100,90)
turtle.fd(200)
turtle.circle(100,90)
You may want to generalize this by creating a function that draws round rectangles with any corner size. The following is the complete code:
import turtle
turtle.speed(0)
turtle.hideturtle()
turtle.setup(1000,1000)
turtle.title('Rounded Rectangle - PythonTurtle.Academy')
turtle.speed(0)
turtle.up()
turtle.hideturtle()
def round_rectangle(center_x,center_y,width,height,cornersize):
turtle.up()
turtle.goto(center_x-width/2+cornersize,center_y-height/2)
turtle.down()
for _ in range(2):
turtle.fd(width-2*cornersize)
turtle.circle(cornersize,90)
turtle.fd(height-2*cornersize)
turtle.circle(cornersize,90)
round_rectangle(0,0,200,300,20)
round_rectangle(-100,300,400,200,100)
round_rectangle(200,-300,300,300,150)
round_rectangle(-200,-300,200,200,50)
It draws the following shape:
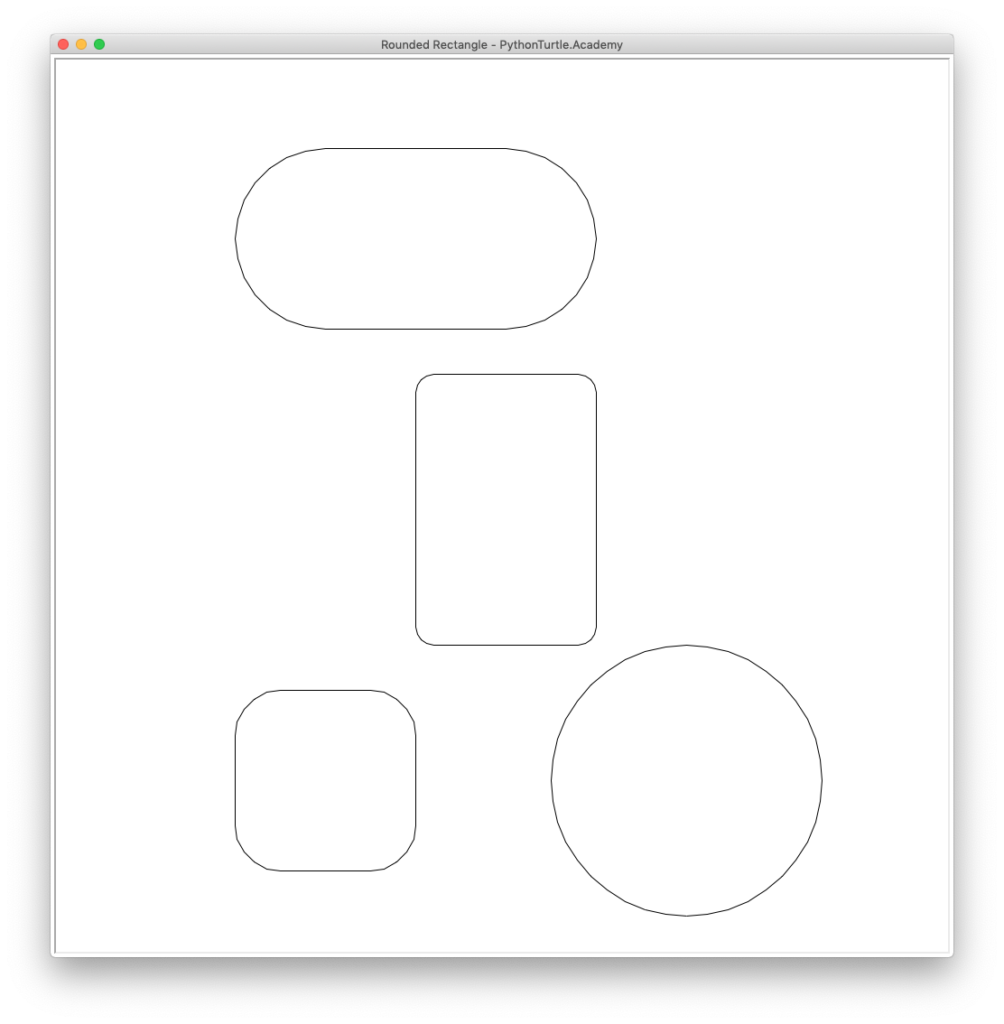
No comments:
Post a Comment